The agent & global objects
A simple guide to help you understand what are the agent and global objects in Phantombuster
First of all, what are these "objects"?
They are small JSON documents stored alongside your agents and Phantombuster account. They are extremely useful for keeping state between different launches of your bots.
Our customers use these objects for many things. One example is for when you want your agent to "remember" where it stopped last time so that it can continue where it left off. For example, you can save the last visited page as a string (URL) or the last page number in a field of type Number.
Agent objects
Every single agent on Phantombuster can have a JSON document associated with it (but other Phantoms can also access it and modify it if necessary).
How to view and edit my Agent object
💡 You can find an Agent object from your dashboard or from a Agent's console. Open its Management menu (three little dots!) and click "Agent object".
Global object
Your Phantombuster account has a single JSON document called the global object. This is useful for keeping state between all of your agents at the same time. The most frequent use we've seen for this object is for storing database connection settings or credentials that have to be shared between agents.
The global one can be used when you want to have a variable available in all your agents, like for example an API key for another service.
How to view and edit my Global object
💡 Head to your global object page to view or edit your global object. This page is also accessible through your developer's page.
In practice
Now how does it work? There are 4 methods provided in the BusterJS module :
const agentObject = await buster.getAgentObject(agentId)
const globalObject = await buster.getGlobalObject()
Agent Id
Not passing an agentId to buster.getAgentObject will return the current agent's result object. You can list your agents using the agent/fetch-all endpoint, or copy the id from the url's path.
The get
method returns the object asked.
No need to put an argument except with getAgentObject()
when you want to get another agent object, the default value will be the ID of the currently running agent.
await buster.setAgentObject(object, agentId)
await buster.setGlobalObject(object)
The set
methods replace the object with the one put as the first argument.
In the case of the agent object, the method doesn't need the agent ID but you can modify another agent's object by specifying it (same as the get
method).
Replace, not update
The set method replaces the current object. If you just want to edit a part of the object, you still have to fetch the full object first then use a set method with the updated JSON document.
Complete example
If you are not familiar with coding on Phantombuster, take a look at our guides, especially on how to manage custom scripts and how to code a custom script.
Here is a simple example of how to use the objects. First, get the object then... set it. That's all 😄
"phantom image: web-node:v1"
"phantombuster flags: save-folder"
/**
* The upper part is phantombuster configuration
* If you would like to know more about script directives:
* @see: https://hub.phantombuster.com/docs/script-directives
*/
const Buster = require("phantombuster")
const buster = new Buster()
;(async () => {
const agentObject = await buster.getAgentObject()
const globalObject = await buster.getGlobalObject()
console.log(`Agent object: ${JSON.stringify(agentObject, null, 2)}`)
console.log(`Global object: ${JSON.stringify(globalObject, null, 2)}`)
agentObject.lastIndex++
globalObject.folderUrl = "http://newurl.com"
await buster.setAgentObject(agentObject)
await buster.setGlobalObject(globalObject)
console.log("\n-------\n")
const newAgentObject = await buster.getAgentObject()
const newGlobalObject = await buster.getGlobalObject()
console.log(`New Agent object: ${JSON.stringify(newAgentObject, null, 2)}`)
console.log(`New Global object: ${JSON.stringify(newGlobalObject, null, 2)}`)
process.exit()
})()
Watch your bot do its job
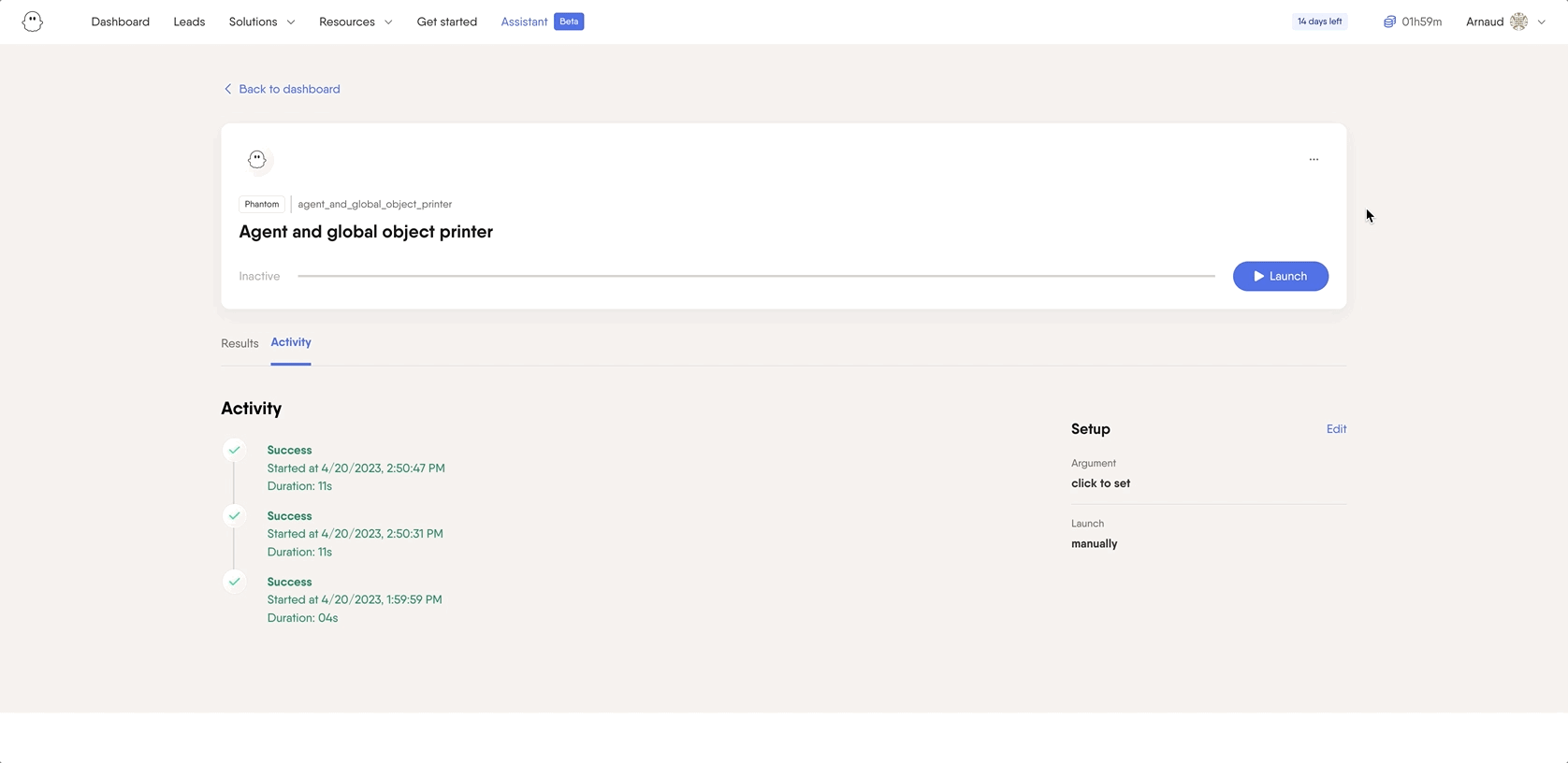
Launch it and enjoy your updated objects!
Updated about 2 years ago